Option Menu:-Android Option Menus are the primary menus of android. They can be used for settings, search, delete item etc.
Here, we are inflating the menu by calling the inflate() method of MenuInflater class. To perform event handling on menu items, you need to override onOptionsItemSelected() method of Activity class.
We are going to see example of option menus. We will see the simple option menus and second, options menus with images.
Required Classes and xml layouts:-
1.MainActivity.java | activity_main.xml
2.Home.java | home.xml
3.menu (create directory inside res with the name menu) | option_menu.xml(right click on menu->new->Menu resource file->give name option_menu.xml->ok. )
Step1:-Add 4 images inside res->drawable with the name (u can add image according to your requirment)
1.home.jpg/png
2.profile.jpg/png
3.about.jpg/png
4.help.jpg/png
Step2:-option_menu.xml
<?xml version="1.0" encoding="utf-8"?>
<menu xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:optionmenu="http://schemas.android.com/apk/res-auto">
<item
android:title="Home"
android:id="@+id/itemHome"
android:icon="@drawable/home"
optionmenu:showAsAction="ifRoom"></item>
<item
android:title="User"
android:id="@+id/itemUser"
android:icon="@drawable/user"
optionmenu:showAsAction="ifRoom"></item>
<item
android:title="About"
android:id="@+id/itemAbout"
android:icon="@drawable/about"
optionmenu:showAsAction="ifRoom"></item>
<item
android:title="Help"
android:id="@+id/itemHelp"
android:icon="@drawable/help"
optionmenu:showAsAction="ifRoom"></item>
</menu>
Step 3:- activity_main
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:gravity="center_vertical"
tools:context="supriya.com.optionmenuexample.MainActivity">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Example Of Option Menu"
android:textSize="30sp"
android:textAlignment="center"
android:textColor="@color/colorAccent"/>
</LinearLayout>
Step 4:-MainActivity.java
Here, we are inflating the menu by calling the inflate() method of MenuInflater class. To perform event handling on menu items, you need to override onOptionsItemSelected() method of Activity class.
We are going to see example of option menus. We will see the simple option menus and second, options menus with images.
Required Classes and xml layouts:-
1.MainActivity.java | activity_main.xml
2.Home.java | home.xml
3.menu (create directory inside res with the name menu) | option_menu.xml(right click on menu->new->Menu resource file->give name option_menu.xml->ok. )
Step1:-Add 4 images inside res->drawable with the name (u can add image according to your requirment)
1.home.jpg/png
2.profile.jpg/png
3.about.jpg/png
4.help.jpg/png
Step2:-option_menu.xml
<?xml version="1.0" encoding="utf-8"?>
<menu xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:optionmenu="http://schemas.android.com/apk/res-auto">
<item
android:title="Home"
android:id="@+id/itemHome"
android:icon="@drawable/home"
optionmenu:showAsAction="ifRoom"></item>
<item
android:title="User"
android:id="@+id/itemUser"
android:icon="@drawable/user"
optionmenu:showAsAction="ifRoom"></item>
<item
android:title="About"
android:id="@+id/itemAbout"
android:icon="@drawable/about"
optionmenu:showAsAction="ifRoom"></item>
<item
android:title="Help"
android:id="@+id/itemHelp"
android:icon="@drawable/help"
optionmenu:showAsAction="ifRoom"></item>
</menu>
Step 3:- activity_main
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:gravity="center_vertical"
tools:context="supriya.com.optionmenuexample.MainActivity">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Example Of Option Menu"
android:textSize="30sp"
android:textAlignment="center"
android:textColor="@color/colorAccent"/>
</LinearLayout>
Step 4:-MainActivity.java
package supriya.com.optionmenuexample; import android.content.Intent; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.Menu; import android.view.MenuItem; import android.widget.Toast; public class MainActivity extends AppCompatActivity { @Override
protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); } @Override
public boolean onCreateOptionsMenu(Menu menu) { getMenuInflater().inflate(R.menu.option_menu,menu); return super.onCreateOptionsMenu(menu); } @Override
public boolean onOptionsItemSelected(MenuItem item) { switch (item.getItemId()){ case R.id.itemHome: startActivity(new Intent(MainActivity.this,Home.class)); Toast.makeText(getApplicationContext(),"Home selected",Toast.LENGTH_LONG).show(); return true; case R.id.itemUser: Toast.makeText(getApplicationContext(),"User Selected",Toast.LENGTH_LONG).show(); return true; case R.id.itemAbout: Toast.makeText(getApplicationContext(),"About Selected",Toast.LENGTH_LONG).show(); return true; case R.id.itemHelp: Toast.makeText(getApplicationContext(),"Help Selected",Toast.LENGTH_LONG).show(); return true; } return super.onOptionsItemSelected(item); } }
Step5:-home.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical" android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center_vertical">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Welcome to Home"
android:textSize="30sp"
android:textAlignment="center"/>
</LinearLayout>
Step 6:-Home.java
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical" android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center_vertical">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Welcome to Home"
android:textSize="30sp"
android:textAlignment="center"/>
</LinearLayout>
Step 6:-Home.java
package supriya.com.optionmenuexample; import android.os.Bundle; import android.support.annotation.Nullable; import android.support.v7.app.AppCompatActivity; /** * Created by Supriya on 9/15/2016. */
public class Home extends AppCompatActivity { @Override
protected void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.home); } }
Output:-
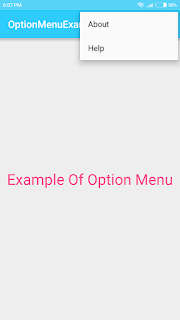
No comments:
Post a Comment